Stacked GitHub PRs
I recently stumbled upon a discussion by @timoliver highlighting the advantages of the “stacked diffs” workflow at Instagram over traditional GitHub Pull Requests. I was intrigued, but a bit confused: this method seems an awful lot like a PR-stacking workflow I use from time to time.
Post by @timoliverView on Threads
The tl;dr is that it’s a recursive twist on the standard feature-branch workflow. Branching off a feature branch works just as well as branching from main
, and GitHub PRs handle this nicely. Just like commits enable you to segment changes within a PR, stacking PRs allows you to segment changes within an overall feature into appropriately-scoped reviews.
It seems like this workflow might not be widely known, so per Tim’s suggestion I decided to dust off the ol’ blog and document how I use it.
Standard PR Workflow
Let me start with my usual PR process, which follows the conventional feature-branch workflow: branch from main, develop, push to remote, and then create a GitHub PR.
I’m a big fan of using commits as checkpoints during development, offering the freedom to experiment with the security of a fallback. For larger PRs, commits can also help reviewers by acting as “mini-PRs”, breaking the overall change down into smaller logical chunks which can be reviewed separately.
In case of conflicts: fetch, rebase on origin/main
, and force-push. (A quick shoutout to IntelliJ’s automatic conflict resolution tool—it’s a lifesaver for rebases!)
After review and approval, squash and merge the changes into main
as a single commit.
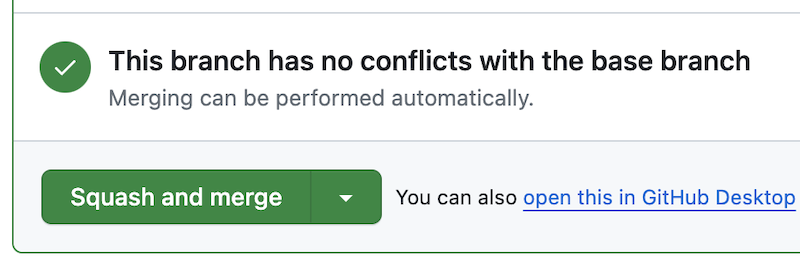
PR Stacking
A potential issue of the above workflow is having to wait for the PR’s review and merge into main
before you can continue development on the changes. PR stacking neatly sidesteps this by branching your next feature branch from the one currently under review, instead of main. Essentially, you’re treating the parent feature branch as your new main
. This recursive approach can be repeated as needed, although I generally don’t exceed one or two levels.
When creating the child PR on GitHub, just set the base to the parent branch (not main
) to avoid mingling changes from the parent. Once the parent PR merges, GitHub automatically updates the child PR’s base to main
(or whatever the parent’s base was), facilitating a smooth, sequential merging of the stacked PRs.
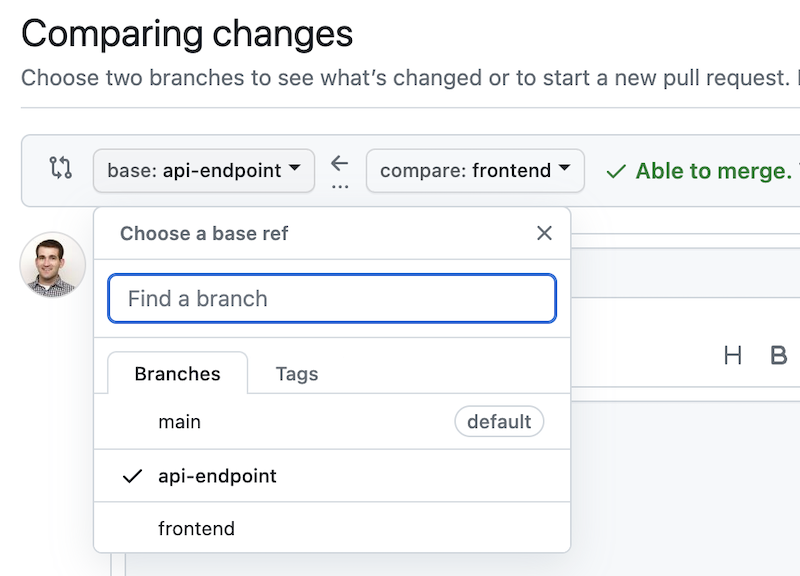
Here’s a quick demonstration using a basic python web app. The overall feature requires a new API endpoint as well as frontend changes, which we will separate into two PRs.
Example
Starting Point
We begin on our main
branch with a simple app.py
file.
app = Flask(__name__)
@app.route('/')
def home():
return render_template_string("<h1>Hello, World!</h1>")
API Endpoint PR
Next we implement the new API endpoint, creating a api-endpoint
branch off of main
and making a commit with the new route.
@app.route('/api/data')
def api_data():
return jsonify({"message": "Hello from API"})
Create a PR on GitHub for the api-endpoint
branch as normal to start the review process.
Stacked Frontend PR
Without waiting for the API PR review, we create a frontend
branch from api-endpoint
. This includes the new API code, which we then utilize in the home
page. After committing, create a PR as usual, but set api-endpoint
as the base, not main
.
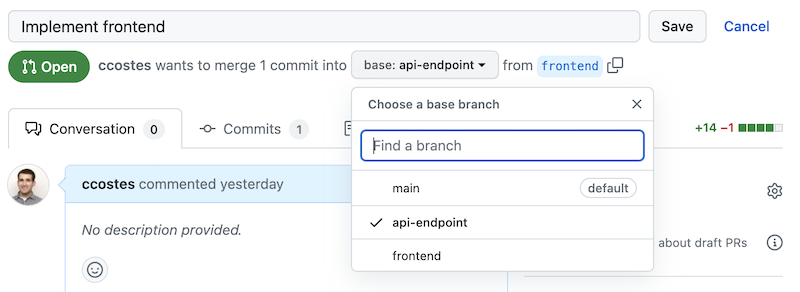
This results in a PR which only includes our frontend changes.
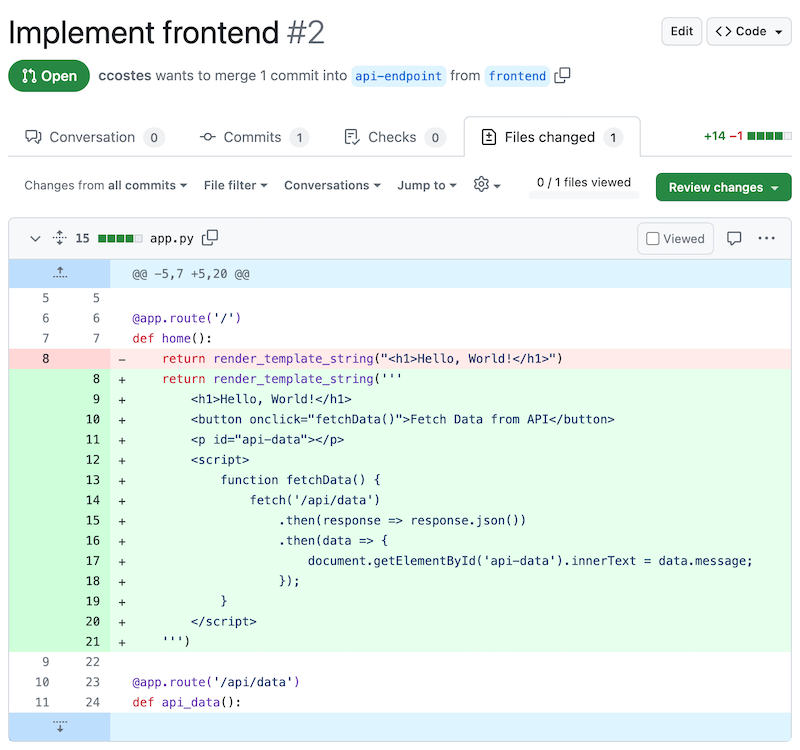
Review and Merge
We now have two independent PRs for review. Post-approval of the API PR, squash and merge it. GitHub will update the Frontend PR’s base to main
. After approval, merge the Frontend PR normally.
Drawbacks
One challenge with PR stacking is rebasing updates from master into child branches. This process mirrors the standard feature-branch workflow, just repeated for every branch starting from the topmost feature branch and repeating for each child. This can become cumbersome with too many child-branches, hence my preference to limit the stacking depth.
Stacked Diffs
I’m interested to learn more about the “stacked diffs” workflow, and tools like Graphite mentioned in the original discussion. It seems similar to the approach described above, but with a PR for each commit.
While smaller PRs quicken review times, you’re also multiplying the number of reviews while also dilute context of the overall change into separate PRs. I worry that this might lead to surface-level feedback and make it harder to review larger design choices that span across commits and might be hard to see by looking at them individually.
That said, it’s always hard to judge tools or processes without first-hand experience, so I’d love to hear from anyone who’s used this workflow in practice!